
Home » News » How To » Raspberry Pi and MCP3008
Raspberry Pi and MCP3008
Read analog signals on Raspberry Pi with MCP3008
hort story: on the Raspberry Pi you can’t analyze analog signals. If you have this need you have to get an external help. The most common configuration involved use of an analog/digital converter (aka ADC, Analog to Digital Converter).
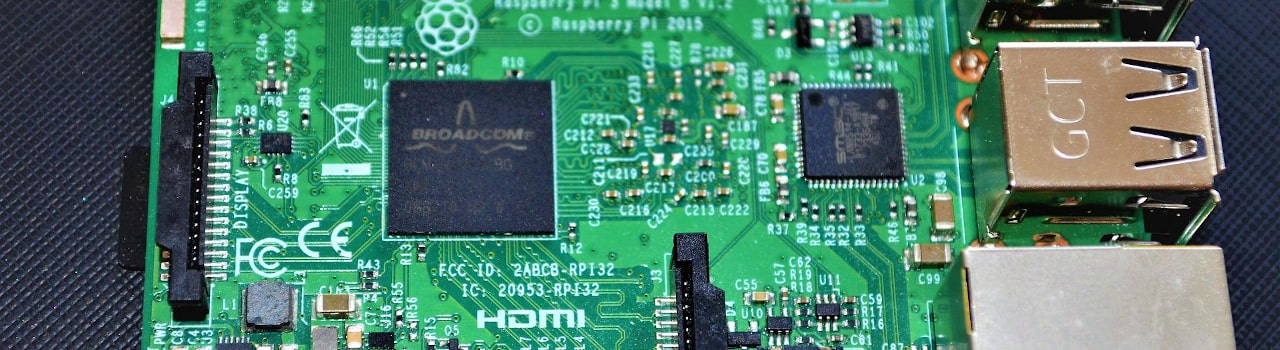
MCP3008
Installing the Development Environment
As a development environment we prefer Python, so let’s open the terminal and install it:
sudo apt-get update
sudo apt-get install build-essential python-dev python-smbus python-pip
sudo pip3 install spidev
Wiring
This way to connect MCP3008 to the Raspberry Pi is called Software SPI.
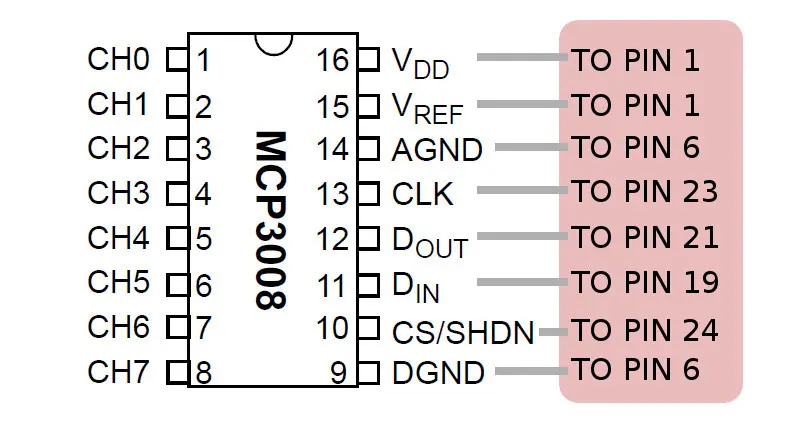
After the wiring, run your Raspberry Pi than execute the follow:
sudo raspi-config
then enable the SPI.
Checking the operation
To check the correct reading from the MCP3008 you can use a simplification of the code suggested by raspibo.org:
import spidev
import time
import math
mcp3008 = spidev.SpiDev(0,0)
adcnum=0 #questo valore identifica il canale da leggere
xferarg=[1,(1+adcnum)<<4,0]
vref = 3.3
ret=mcp3008.xfer2([1,(8+adcnum)<<4,0])
adcout = (((ret[1]&3) << 8) + ret[2]) * 3.3 / 1024
print ((ret[1]&3) << 8) + ret[2], adcout
mcp3008.close()
Adafruit’s library
You can take advantage of the Adafruit library to query the MCP3008 in a humanly understandable way. You can install the library with the following command:
sudo pip install adafruit-mcp3008
fter that you can try this code (it’s a simplification of Tony DiCola’s simpletest.py) to check the ADC:
import time
import Adafruit_GPIO.SPI as SPI
import Adafruit_MCP3008
CLK = 18
MISO = 23
MOSI = 24
CS = 25
mcp = Adafruit_MCP3008.MCP3008(clk=CLK, cs=CS, miso=MISO, mosi=MOSI)
print('Reading MCP3008 values, press Ctrl-C to quit...')
print('| {0:>4} | {1:>4} | {2:>4} | {3:>4} | {4:>4} | {5:>4} | {6:>4} | {7:>4} |'.format(*range(8)))
print('-' * 57)
while True:
values = [0]*8
for i in range(8):
values[i] = mcp.read_adc(i)
print('| {0:>4} | {1:>4} | {2:>4} | {3:>4} | {4:>4} | {5:>4} | {6:>4} | {7:>4} |'.format(*values))
time.sleep(0.5)
DO YOU WANT OUR CONSULTING?
Discover some of our products
Caronte Consulting designs and manufactures hardware and software systems in the field of Industry 4.0, equipped with a sophisticated and effective artificial intelligence.
Our devices are made to measure to the customer’s needs and make it possible to completely automate industrial processes, optimizing production and creating a saving of raw materials and energy consumption.